I am going to explain how to start programming in java. Lets write a simple program to print something.
for that you need to get a text editor. If you are a windows user, you can use the Notepad.
this is notepad
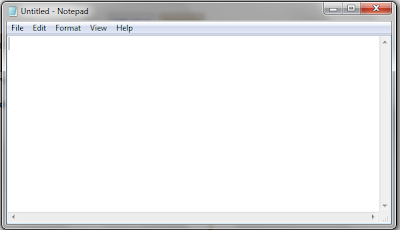
now you can start to type the program.
first of all we need to create a java class. All the java coding should implement under this class.
This is the way of creating a class,
class MyFirstClass{
}
"class" is a keyword. Keywords should be have correct spelling and correct upper and lowercase. (java is a case sensitive language).
"MyFirstClass" is the name of the class that we are going to create. It can have any name. In good coding practices, we start class name with a capital letter and we should always try to use meaningful names as class names.
A java class is same as a factory.From now I am going to explain java coding while comparing it with a tea factory. It will be a good help for you to understand java easily.
In a tea factory there is a building. All the raw materials and all the machines are inside the building. In a java class, all the attributes and all the methods should be implemented inside the class.
In a factory there is a main machine. And also in a java class, there should be a main method.
What is a method??A method is like a machine of a factory. A machine is used to produce something. A method is also used to produce something.
For a machine there should be raw materials to produce something (Some machines do not need raw materials). In a method there should be variables to produce something.(some methods do not use variables).
For a machine there should be two doors. One for to give inputs(raw materials) and the other one to get the output. In a method, there should have two doors to give inputs and get outputs.
example for a method,
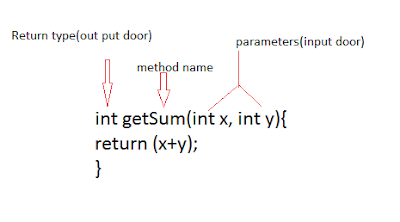
About return types and additional details of method will be discussed in later. Main method is a different method. It is used to handle all other methods like main machine controls all the other machines in a factory. Instead of that processing also can be done inside the main method.
Main methodMain method should be like this,
public static void main(String args[]){
}Now lets move to the our topic(Start Programming with java) again.
To get successful output, there should be a main method inside of a class.(for this beginner level). I am going to print some text using a java code. I am going to use main method to do this process rather than using additional methods. Because this is the our first program.
class MyFirstClass{
public static void main(String args[]){
System.out.println("hello.. world");
}
}
This is the java code for our first program. I used main method to print the "hello.. world" sentence.
Write this code on a notepad and save it as a .java file.

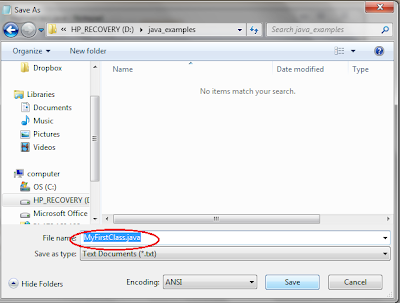
I saved the file in D:\java_examples directory.
Now I want to compile the java class. In the compiling process compile errors of the code, are captured.(Eg spelling mistakes).
To compile the class,
first I should open the command prompt.
To open the command prompt go to start and type cmd one the search bar and press enter(I am a windows 7 user)
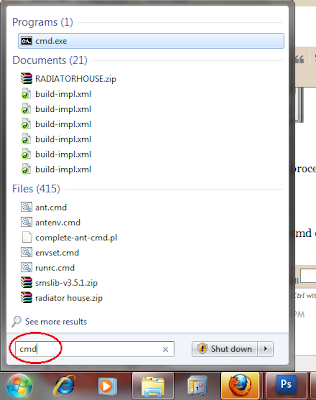
then you can see the command prompt like this
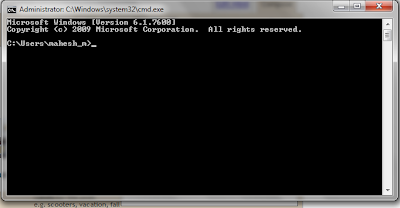
To compile the java code, we should go to the folder in which the java class is saved. So I should go to "java_examples" folder inside the D partition.
For that type this commands on the command prompt.
If your folder is in the D partition you can type
d: and press enter.
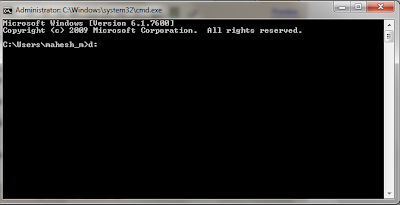
After press enter you can see the command prompt like this,
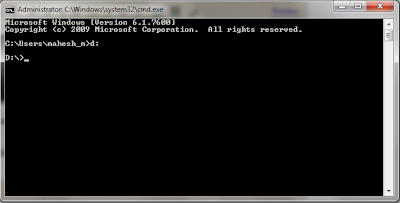
Now we are in the D partition of the computer.
Then we have to go to the folder which is having my java class. For that, type
cd folder name and press enter
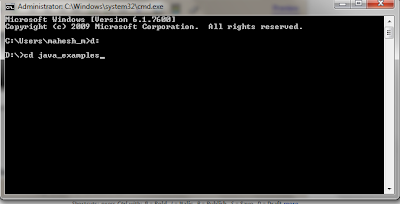
After press enter, command prompt can be seen like this,
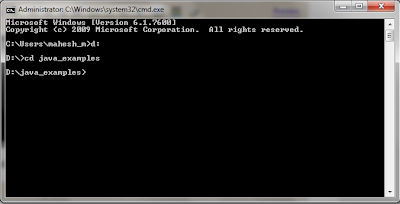
Now we are inside the "java_examples" folder.
Then we should give a command to compile our class.
Type
javac file name.java and press enter
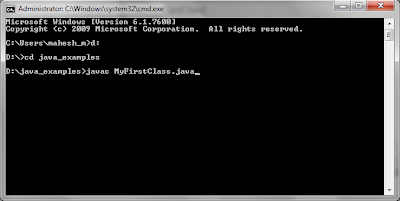
After press enter, if your code doesn't have any compile errors it will give this kind of display on the command prompt.
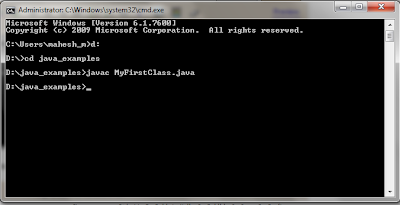
Now we have finished the compilation process. Then we have to run our program. For that we should type
java class name and press enter
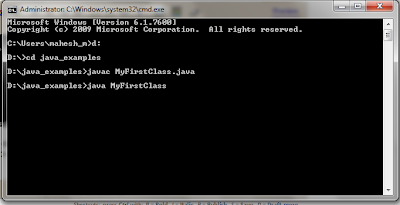
After pressing enter, you can see the output of our program like this,
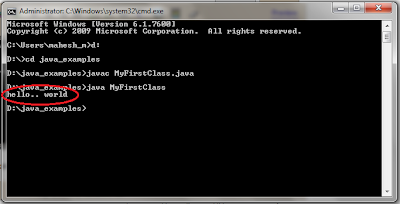
Thats all.. :) :) :)